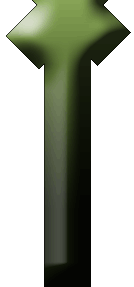 |
Botbattle Programming Tutorial v2.0 (revised by Tangent Man)
Suggest opinions, bugs and more here
PROGRAM BASICS
A bot program (like any other program) is made up of a set of commands, which are interpreted, in part,
with the Instruction Pointer. The Instruction Pointer (IP) begins at the beginning of your bot's code
when the match starts, and scrolls through your code, one instruction at a time. There are about 15
frames per second during game play, and for each game frame your bot can execute up to 100 instructions.
If your bot executes 100 instructions, a movement or fire command then his turn is over for that game frame.
THE MAIN FUNCTION
The primary section of your program is the "main" function. For very basic bots (novice-bots), all your
code could be there (between 'main:' and ':main' in the sample below).
main:
set abot to closest bot
set target to abot
if
bullet too close
fire item4
endif
fire item1
move target
move target
fire item2
:main
end
HOW TO DO IF/ELSE STATEMENTS
The way to determine which code to execute is through the use of IF/ELSE statements. IF/ELSE statements
are decision-makers.
For example:
If I am hungry
eat a pizza
else
watch a video
endif
Currently, you can NOT nest one IF statement within another, but you can call functions from within other
functions (which brings us to the next topic)...
FUNCTIONS
While you could write one long program, it is advised that you break your code into discreet, reusable
pieces, called functions. Your bots brain can consist of up to 700 lines of code, but remember that only
100 are executed each frame, and functions allow only the necessary code to be executed. A function is
used when you have a small piece of code that you would like to use in a number of different places, or as
a method of executing a series of decisions (this is how you nest IF statements). You can write functions
to do just about anything:
- detect enemies
- set a target
- fire a weapon
- run away
- check your health
A Function is like a short program, but its code is seperate from the 'main' function, and can be
identified by the colon (:) after its name. You call a function from within the main (or another)
function simply by entering its name (the same as you would any command). In the following example, the
function names 'targetabot' is called from within main. When the function's code has been executed
(when it reaches the 'return' statement, the IP jumps back to where the function was called from:
main:
targetabot
:main
targetabot:
set abot to closest bot
set target to abot
return
end
CODE SAMPLE
In this example, the following code checks if the powerup is on and if it is, moves three moves towards it.
If not, it targets a bot, moves towards it, and fires a bullet at it. (notice the use of IF/ELSE
statements and Functions):
main: <- beginning of the program
if <- IF statement
powerup on <- the condition of the IF statement
getpowerup <- function call
else <- ELSE part of the IF statement
killabot <- another function call
endif <- the end of the IF/ELSE statement
main <- the end of the main function
(but not the end of the program)
getpowerup: <- this is the beginning of a function
set direction to powerup (note the colon at the end of the name)
move (this is how you define it as a function)
move
move
return <- this is the end of the function
killabot: <- this is the beginning of another function
set abot to closest bot
set target to abot
set direction to abot
move
move
shootabot <- another function call
return (just use the function name without the colon ':')
shootabot: <- yet another function
if <- here is an IF statement within a function
target is medium range
fire item2
endif
if <-here is another
target is close range
fire item1
endif
return
end <- this marks the end of the program
To see a list of bot commands, click here
Suggest opinions on bettering this tutorial here
To see parts of bot code click here
|
 |